ASP.NET - Login and SignUp with LinkedIn API
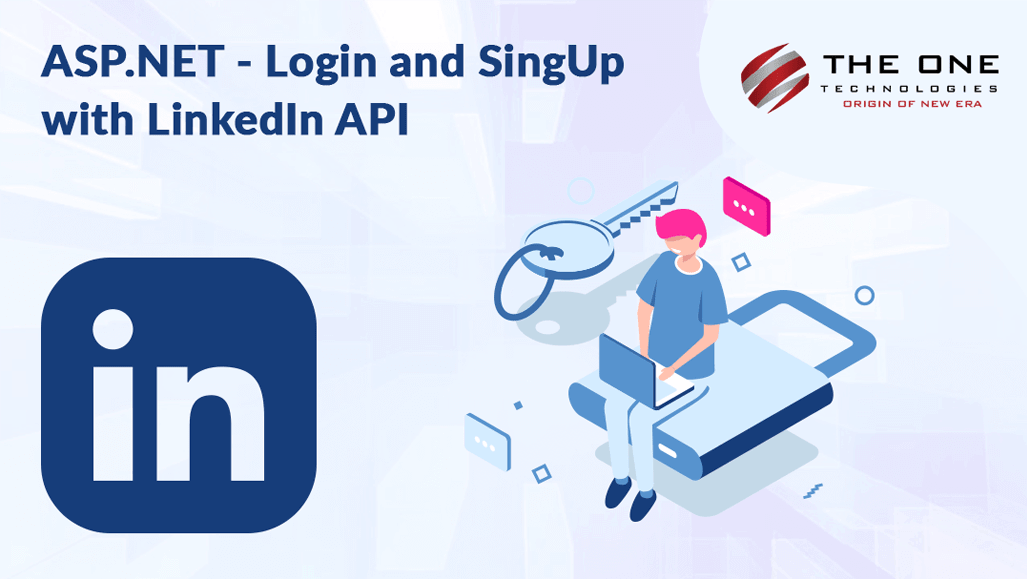
In our previous blog post we looked at Login with Google plus using Google Plus API in asp.net.
Here I will explain how to integrate SingIn with LinkedIn button to asp.net web application using JavaScript API. By extending scope, we can also use this same functionality for Sign Up with Linkedin account or Linkedin login authentication for website in asp.net.
Obtain LinkedIn API key
The first step involves creating an application into LinkedIn developer portal.
- Once you login to developer portal, you can find API Keys link by hovering over your name at top right corner.
- Clicking on 'API Keys' link, it will list out your LinkedIN application if you have created any.
- Click on 'Add New Application' link, which shows the form to input different information about your application.
- In Default scope section, checkmark the scopes related to information you want to gather from the user.
- In 'Javascript API domains' section: please input comma separated domains on which you are going to run this application.
- Click 'Add Application' button. Once application is created you can see API Key and Secret Key for that application. These keys will be needed in our asp.net code to talk with LinkedIn API.
Create an asp.net class to hold deserialized JSON data from LinkedIn
public class LinkedinUser
{
public string emailAddress { get; set; }
public string firstName { get; set; }
public string id { get; set; }
public string lastName { get; set; }
public string pictureUrl { get; set; }
}
ASP.Net login page to show SingIn with LinkedIn button
- In Head section of the page, reference the LinkedIn API javascript file.
- Inside body, place the <script type="in/login" ... tag which automatically shows the button. Notice the data-onAuth="onLinkedInAuth" attribute, which is the callback function.
- Write Javascript function onLinkedInAuth to handle the SignIn callback we get as a result from LinkedIn signin dialog.
<head>
<title></title>
<script type="text/javascript" src="http://platform.linkedin.com/in.js">
api_key: YOUR_API_KEY_HERE
authorize: false
credentials_cookie: true
</script>
</head>
<body>
<div id="linkedin_login"><script type="in/login" data-onAuth="onLinkedInAuth"><a href="#" onclick="location.reload();">Reload Page</a></script></div>
<script type="text/javascript">
//LinkedIn Auth Event
function onLinkedInAuth() {
var loc = 'callback.aspx?accessToken=' + IN.ENV.auth.oauth_token;
window.location.href = loc;
}
//End LinkedIn
</script>
</body>
Code for callback.aspx
As we passed the access token from the first page in querystring, we can use that here to make an API call to LinkedIn oauth/accessToken method to retrieve the user's token and token secret. Which can be used in sub-sequent API calls.
public partial class callback : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(Request.QueryString["access_token"])) return;
WebUtility oAuthLi = new WebUtility("linkedin");
string response = oAuthLi.oAuthWebRequest(WebUtility.Method.POST, "https://api.linkedin.com/uas/oauth/accessToken?xoauth_oauth2_access_token=" + oAuthLi.UrlEncode(Request.QueryString["accessToken"]), "");
string[] tokens = response.Split('&');
string token = tokens[0].Split('=')[1];
string token_secret = tokens[1].Split('=')[1];
//STORE THESE TOKENS FOR LATER CALLS
oAuthLi.Token = token;
oAuthLi.TokenSecret = token_secret;
//SAMPLE LINKEDIN API CALL
string url = "http://api.linkedin.com/v1/people/~:(id,first-name,last-name,email-address,picture-url)?format=json";
string json = oAuthLi.oAuthWebRequest(WebUtility.Method.GET, url, "");
JavaScriptSerializer js = new JavaScriptSerializer();
LinkedinUser linkedinUser = js.Deserialize<LinkedinUser>(json);
if (linkedinUser != null)
{
Response.Write("Welcome, " + linkedinUser.emailAddress);
}
}
}
If you are looking for expert and talented ASP.Net Programmers to integrate Linked APIs into your existing website contact us to hire dot net developer.