ASP.NET - Signin with Google plus using Google Plus API - Part 2
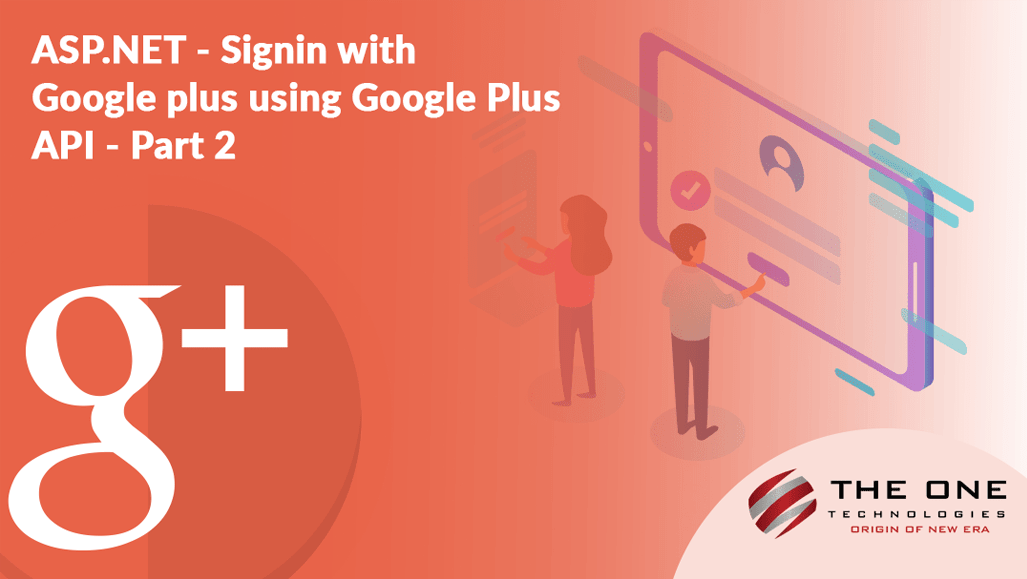
In this post, we are going to use clientId obtained from google API console and create an asp.net signin page to use user's google plus email. Lets first create few basic classes which we can use to deserialize the JSON responses we get from google api calls.
Classes to hold deserialized JSON data from Google Plus
public class GoogleEmail
{
public Data data { get; set; }
}
public class Data
{
public string email { get; set; }
}
Login page to show Google+ Login button
This page holds the Google Plus Sign In button, clicking on which user will be presented with Google Plus Signin dialog screen.
- In Head section of this page place the reference of plusone api javascript file.
- Inside body place the button with google+ client api attributes. Be sure to use your Application ClientId for the data-clientid attribute.
- Javascript function to handle the SignIn callback we get as a result from Google+ signin dialog. If user authenticates with google+ successfully, we will get an Access Token. We pass this access token to our second asp.net page.
<head>
<script type="text/javascript">
(function () {
var po = document.createElement('script');
po.type = 'text/javascript'; po.async = true;
po.src = 'https://plus.google.com/js/client:plusone.js';
var s = document.getElementsByTagName('script')[0];
s.parentNode.insertBefore(po, s);
})();
</script>
</head>
<body>
<div id="gConnect">
<button class="g-signin"
data-scope="https://www.googleapis.com/auth/plus.login https://www.googleapis.com/auth/userinfo.email "
data-requestvisibleactions="http://schemas.google.com/AddActivity"
data-clientid="[YOUR APPLICATION CLIENT ID HERE]"
data-accesstype="offline"
data-callback="onSignInCallback"
data-theme="dark"
data-cookiepolicy="single_host_origin">
</button>
</div>
<script type="text/javascript">
/**
* Calls the helper method that handles the authentication flow.
*
* @param {Object} authResult An Object which contains the access token and
* other authentication information.
*/
function onSignInCallback(authResult) {
if (authResult['access_token']) {
// The user is signed in
var loc = '/callback.aspx?accessToken=' + authResult['access_token'];
window.location.href = loc;
} else if (authResult['error']) {
// There was an error, which means the user is not signed in.
// As an example, you can troubleshoot by writing to the console:
alert('There was an error: ' + authResult['error']);
}
//console.log('authResult', authResult);
}
</script>
</body>
Code for callback.aspx
As we passed the access token from the first page in query string, we can use that here to make an API call to Google+ userinfo/email method to retrieve the user's email address. Using JavaScript Serializer class we can deserialize the json string into the .net classes we created in first step.
public partial class callback : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(Request.QueryString["access_token"])) return;
//let's send an http-request to Google+ API using the token
string json = GetGoogleUserJSON(Request.QueryString["access_token"]);
//and Deserialize the JSON response
JavaScriptSerializer js = new JavaScriptSerializer();
GoogleEmail oUser = js.Deserialize<GoogleEmail>(json);
if (oUser != null)
{
Response.Write("Welcome, " + oUser.data.email);
}
}
/// <summary>
/// sends http-request to Google+ API and returns the response string
/// </summary>
private string GetGoogleUserJSON(string access_token)
{
string url = "https://www.googleapis.com/userinfo/email?alt=json";
WebClient wc = new WebClient();
wc.Headers.Add("Authorization", "OAuth " + Request.QueryString["accessToken"]);
Stream data = wc.OpenRead(url);
StreamReader reader = new StreamReader(data);
string retirnedJson = reader.ReadToEnd();
data.Close();
reader.Close();
return retirnedJson;
}
}
If you are looking for expert and talented ASP.Net Programmers to integrate different social media APIs into your existing website contact us to hire .net developers.