Create CI/CD Pipeline for Node JS application on Gitlab
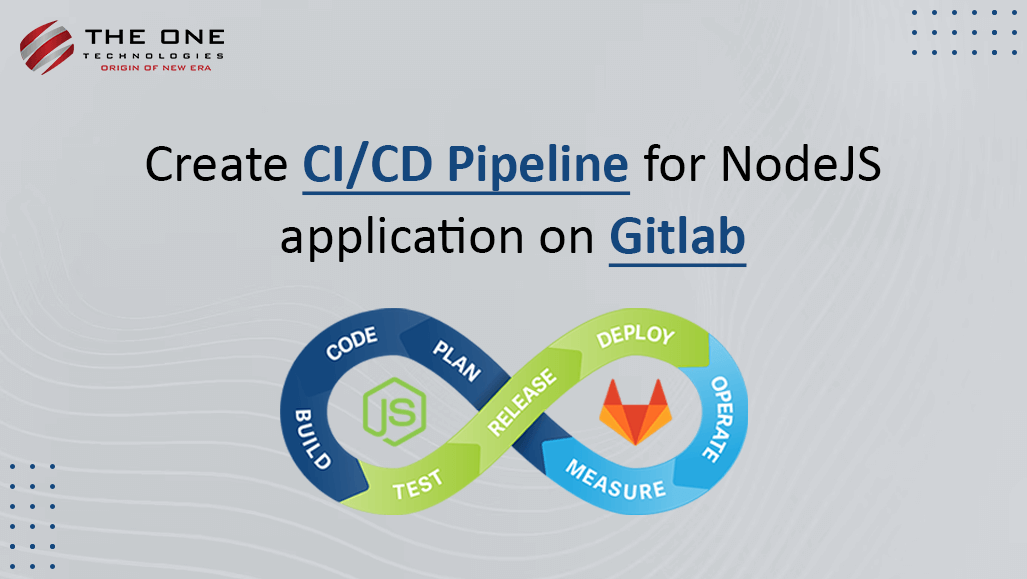
Nowadays, everyone desires automation processes. For example, when we develop our REST APIs in Node.js, if you need to deploy them on an AWS EC2 instance, you could do it manually. However, how often do you want to repeat this manual deployment? Instead of manual deployment, we can implement a CI/CD pipeline that automatically handles this each time you merge code into your deployment branch.
Table of Contents
- Create Sample Node JS application
- Publish code on Gitlab
- Write CI/CD pipeline
- Setup Environment variables
- Set variable on Gitlab
- Conclusion
- People Also Ask
Create Sample Node JS application
Here, we create one node js application which will return a json object.
// Import required modules
const express = require('express');
// Create an Express application
const app = express();
const port = 3000;
// Define a route that returns a JSON object
app.get('/api/sample', (req, res) => {
// Define your sample JSON object
const jsonObject = {
message: 'This is a sample JSON object',
data: {
name: 'Aditya Patel',
age: 30,
email: 'aditya.patel@example.com'
} };
// Send the JSON object as response
res.json(jsonObject);
});
// Start the server
app.listen(port, () => {
console.log(`Server is listening at http://localhost:${port}`);
});
Publish code on Gitlab
Create account on Gitlab
Commands to publish or push your code.
- git init
- git add remote origin http://gitlab.com/test-node-js.git
- git add .
- git commit –m “Initial server created in Node JS.”
- git push origin
Write CI/CD pipeline
stages:
- test
- deploy
test:
stage: test
before_script:
- apt-get update -qy && apt-get install -y rsync && apt-get install -y openssh-client
- mkdir ~/.ssh
- echo "$AWS_SECRET_ACCESS_KEY" > ~/.ssh/id_rsa
- chmod 600 ~/.ssh/id_rsa
- ssh-keyscan -H $AWS_PUBLIC_IP >> ~/.ssh/known_hosts
script:
- rsync -avz --delete --exclude='.git/' . $AWS_USER_NAME@$AWS_PUBLIC_IP:/var/www/temp
- ssh $AWS_USER_NAME@$AWS_PUBLIC_IP 'cd /var/www/temp && npm install'
deploy:
stage: deploy
needs:
- test
before_script:
- apt-get update -qy && apt-get install -y rsync && apt-get install -y openssh-client
- mkdir ~/.ssh
- echo "$AWS_SECRET_ACCESS_KEY" > ~/.ssh/id_rsa
- chmod 600 ~/.ssh/id_rsa
- ssh-keyscan -H $AWS_PUBLIC_IP >> ~/.ssh/known_hosts
script:
- rsync -avz --delete --exclude='.git/' . $AWS_USER_NAME@$AWS_PUBLIC_IP:/var/www/dev
- ssh $AWS_USER_NAME@$AWS_PUBLIC_IP 'cd /var/www/dev && pm2 stop app && npm install && pm2 start app'
Note: Here, I am using docker runner in Gitlab to run the jobs.
Setup Environment variables
- $AWS_USER_NAME
- $AWS_PUBLIC_IP
- $AWS_SECRET_ACCESS_KEY
Examples:
- $AWS_USER_NAME: aditya
- $AWS_PUBLIC_IP: 13.256.55.256
- $AWS_SECRET_ACCESS_KEY (This is .pem file which will you get from AWS Dashboard.)
Example value of .pem file is:
-----BEGIN RSA PRIVATE KEY-----
MIIEpAIBAAKCAQEAmVOrpe2UrfSs4/LAgdqRo6RwWfb+hWLzpsiye+FtPn0K8zyh
LGfZdU5nT9fBh9U31wRyNWOeCZvQwpEXWKfLKGmfXX4Ot2Sd98qfeEMz+kUVgMRi
KEhHOXEbZgp8z95esBV5MzDFWd5cMnIiajJefnRNK7I7zUpG6332pnNf62qWxRUG
fwfjtxvo3Os3VJXkK34zrhqavSFz5OvTBDqIJzOL4/8UXnXbb53baTLo2E3k6Co/
yBAiPj0UcNF+KAEIujgCXq1UDwRIkMYYLuhG9CZi0Ehk4ebG+b4KqnKQQ31FwhGq
b+OyXlFM5HLLPv8mTx1km7K2sEfpeRvnnvz/OQIDAQABAoIBAF/u3w4Bma5B0c8J
+vMEe21lHLrddKCmqgZnum0SPdUETc6k17899uyTssZdG4TOnpKVAJRxENe4OyUR
vQbGbbGKbKEFl1WdyczZZW/MtH/Zq7uWpEfJ+W5yW4877p3s51fLB7tEv6YcH5mc
mfXSMBd00zgM26f12mvLs4Cv3YsgfLxqmfO+NM43EXUrpOTTPEWs33QYD1gymnwI
-----END RSA PRIVATE KEY-----
Set variable on Gitlab
Go to your repository then navigate to Settings > CI/CD > Variables
Congratulations 🥂 🥳 you’ve successfully automated your deployment process.
Conclusion
By employing this process, we reduce our reliance on human resources and avoid wasting time on deployments, allowing us to focus on other tasks. Also, it promotes team organization and provides a more effective approach for project managers to align things accordingly.
So, are you looking to enhance productivity and team collaboration? Then partner with a leading mobile app development company like The One Technologies to streamline your processes and achieve project alignment efficiently!
People Also Ask
What is a CI/CD pipeline?
A CI/CD pipeline automates the process of building, testing, and deploying software. It promotes dependable and quick software delivery while aiding in the maintenance of code quality.
What are the basic stages in a Node.js CI/CD pipeline?
A typical Node.js CI/CD pipeline includes stages such as:
- Build: Install dependencies and build the application.
- Test: Run automated tests to ensure code quality.
- Deploy: Deploy the application to a staging or production environment.
How do I trigger the CI/CD pipeline on GitLab?
When you push changes to your repository on GitLab, the CI/CD pipeline gets started instantly. A pipeline can also be manually started using the GitLab API or the GitLab UI.
Why should I use a CI/CD pipeline for my Node.js application?
By automating repetitive operations, a CI/CD pipeline increases team productivity by reducing manual errors during deployment, ensuring consistent and dependable software delivery, and improving overall team performance.
What are the benefits of implementing CI/CD for my development process?
By automating testing, CI/CD implementations increase code quality, shorten time-to-market for new products, foster better teamwork, and facilitate quicker feedback loops.
Will setting up a CI/CD pipeline require a lot of initial effort?
Configuring a CI/CD pipeline requires some initial setup, but the time savings, decreased errors, and enhanced project management that result over time make the work worthwhile.
Author Bio
Aditya Patel is an Associate Software Engineer at The One Technologies, specializing in web and mobile app development. He embarked on his IT journey in 2022 during his college years, diving into web technologies such as HTML, CSS, and JavaScript. Passionate about leveraging technology for societal good, Aditya is particularly drawn to projects benefiting farmers that streamline agricultural processes and improve livelihoods. Looking ahead, he aspires to establish his own IT company, committed to user-centric design and fostering a positive societal impact.