Large Language Models (LLM) in AI & Integrating LLM with .NET Core
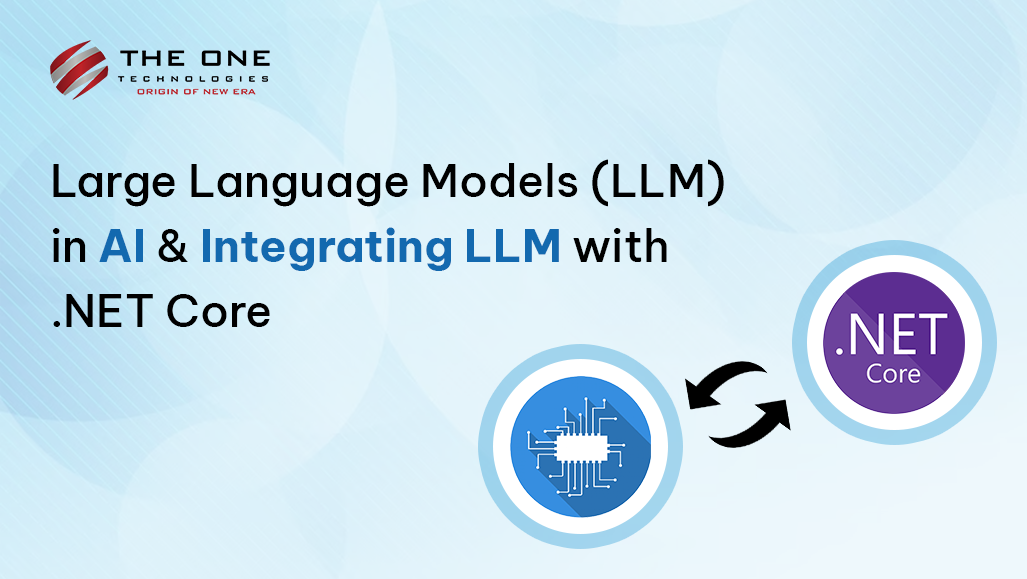
Introduction
LLMs are part of artificial intelligence models that interpret and generate human-like text. These models have acquired considerable notice because of their extraordinary precision in understanding and producing natural language. LLMs are trained on a large quantity of textual material, which allows them to recognize spoken language nuances as well as context, syntax, and pattern detection. Facebook's RoBERTa, Google's BERT (Bidirectional Encoder Representations from Transformers), and OpenAI's GPT (Generative Pre-trained Transformer) series are popular instances of LLMs.
Table of Content
- Introduction
- Key features of LLMs
- Applications of LLMs
- Integration of LLMs with .NET Core
- Considerations
- Conclusion
Key Features of LLMs
1. Natural Language Understanding: LLMs are pretty good for positions that involve question answering, sentiment analysis, and language translation because of their proficiency in understanding and interpreting human language.
2. Text Generation: These models enable applications like chatbots, content creation, and code generation by producing coherent and contextually relevant text.
3. Context Awareness: Longer text inputs are maintained in context by LLMs, facilitating more insightful exchanges and replies.
4. Fine-tuning: Although LLMs are pre-trained on many datasets, their performance can be enhanced by adjusting them for specific applications or domains.
Applications of LLMs
- Customer Support: LLM-powered chatbots and virtual assistants can improve customer service efficiency by instantly responding to their inquiries.
- Content Creation: Create blog posts, articles, and marketing copy automatically using AI-generated material that complies with predetermined standards.
- Language Translation: Translate text between languages fluently and accurately to promote international communication.
- Code Assistance: Help developers by producing code snippets, offering enhancement suggestions, and answering questions about code.
Integration of LLMs with .NET Core
Leveraging AI's capabilities in your apps is made possible by integrating LLMs with .NET Core. The cross-platform and adaptable.NET Core framework offers a stable environment for creating contemporary apps. This is a detailed tutorial on how to integrate LLMs with .NET Core:
Step 1: setup the.NET Core project
1. Create a new .NET core project: Start by creating a new .NET Core project using the following command:
dotnet new console -n LLMIntegration
cd LLMIntegration
The hub in this example contains a SendMessage method that sends out a message to every client that is connected.
2. Install required NuGet packages: Add necessary NuGet packages to facilitate communication with LLM APIs. For example, you might use RestSharp for making HTTP requests:
dotnet add package RestSharp
Step 2: Choose an LLM API
Choose a Large Language Model API to integrate with your .NET Core application. Popular options include:
- OpenAI GPT: Access OpenAI's models via the OpenAI API.
- Hugging face transformers: Use pre-trained models from the hugging face model Hub.
Step 3: Implementation of LLM Integration
1. Create an API Client: Implement a class to interact with the chosen LLM API. For instance, using the OpenAI GPT API:
using RestSharp;
using System.Threading.Tasks;
public class OpenAIClient
{
private readonly RestClient _client;
private readonly string _apiKey;
public OpenAIClient(string apiKey)
{
_apiKey = apiKey;
_client = new RestClient("https://api.openai.com/v1/");
}
public async Task<string> GenerateText(string prompt)
{
var request = new RestRequest("completions", Method.Post);
request.AddHeader("Authorization", $"Bearer {_apiKey}");
request.AddJsonBody(new
{
model = "text-davinci-003",
prompt = prompt,
max_tokens = 100
});
var response = await _client.ExecuteAsync(request);
return response.Content;
}
}
2. Use the API Client: Utilize the API client in your application to generate text using LLMs.
class Program
{
static async Task Main(string[] args)
{
var apiKey = "YOUR_OPENAI_API_KEY";
var openAIClient = new OpenAIClient(apiKey);
string prompt = "What is the capital of France?";
string result = await openAIClient.GenerateText(prompt);
Console.WriteLine("AI Response: " + result);
}
}
Step 4: Run your application
Compile and run your .NET Core application to see the LLM integration in action:
dotnet run
Considerations
- API Key Security: API keys must be stored securely and not hardcoded into your application code. Use environment variables or a secure vault for storage.
- Rate Limits and Costs: Be aware of any rate limits or costs associated with using LLM APIs. Optimize requests to stay within usage limits and budget.
- Error Handling: Implement error handling to gracefully manage API failures or unexpected responses.
Conclusion
LLMs are transforming how software interacts with spoken language, opening new possibilities for automation and improved user experiences. By integrating them with .NET Core, developers may leverage artificial intelligence in their applications, creating opportunities for creative solutions in a range of industries. You can easily incorporate LLMs into your .NET Core projects and realize the full potential of AI-driven text production and processing by following the instructions provided in this blog.
Author Bio
Fenil Lathigra is an Associate Software Engineer at The One Technologies. Throughout his career, he has learned various new technologies and tools used in web development. Fenil is currently focused on exploring trending technologies to further expand his knowledge. His professional goal is to work on significant projects and continually enhance his skills.