Middleware in .NET Core Application
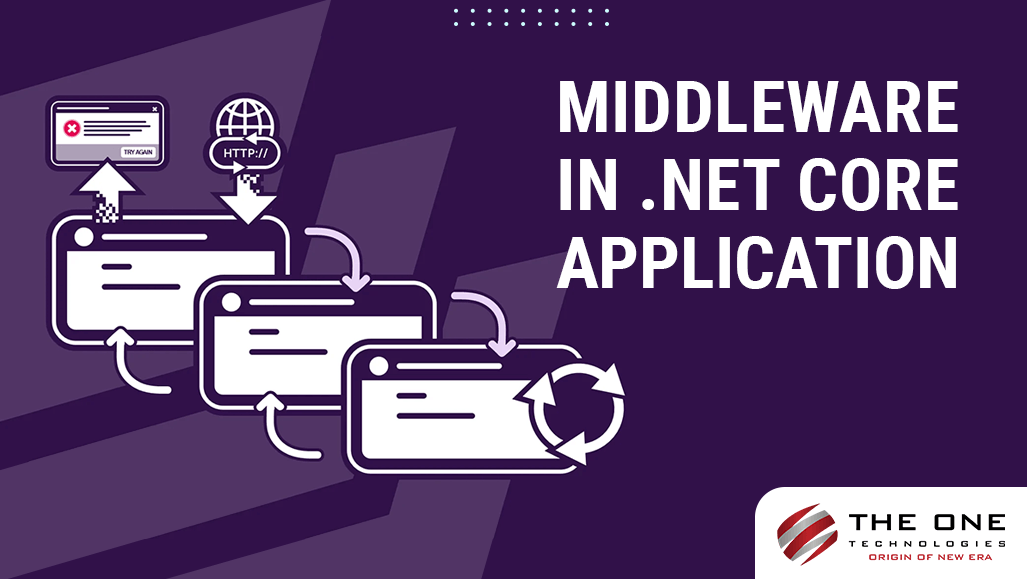
Hello, .NET Developers, so you all are aware of the middleware in .NET core application.
In the world of .NET Core, middleware acts like a backstage magician, managing the flow of requests and responses through a dynamic pipeline, making sure that your application dazzles with speed and precision.
Table of Contents
What is Middleware?
Middleware is a component that executes automatically with every request. .NET Core offers built-in middleware, lets you add more through NuGet packages, and even creates your custom middleware.
Basically, it is one type of filter request in a web application context. Middleware can be used for different purposes, including request filtering. It can filter requests based on various criteria. E.g. you might have middleware that checks whether a user is authenticated before allowing access to certain URLs or routes. By examining these attributes of incoming requests, middleware can decide whether to allow, reject, modify, or redirect them before passing them on to the main application logic.
Each middleware executes sequentially, collectively handling incoming requests in the specified order.
In older versions of .NET Core, middleware configuration was done in the Startup.cs file.
However, in later versions, such as .NET 6.0 and beyond, the Startup.cs file was removed. Instead, middleware configuration and other application setup tasks were combined into the Program.cs file for improved organization and clarity.
Let’s create a .NET core application
Create a .NET Core project
In the .NET Core project, the folder structure is organized with the Startup.cs file containing the Configure method, which includes configurations for several built-in middleware components.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
Middleware ordering
Middleware components execute in the order they are added. It's like creating a to-do list for your application, making sure each task is done at the correct time and in the proper sequence.
The order matters because each middleware plays a specific role and affects the outcome of the response.
The first middleware received the request, updated it as needed, and then gave control to the next middleware. Similarly, the first middleware executes at last while processing the response. That's why it's important to have error handlers (Exception handler middleware) first in the process they can catch any problems and show them in a way that's easy for the user to understand.
Custom middleware
We are going to cover,
- How to create custom Middleware?
- How to use custom middleware in the HTTP pipeline?
Although ASP.NET has plenty of pre-made middleware, there are times when we have to create custom middleware to fulfill our unique needs.
Here we are creating custom middleware for logging requests, so we don’t need to write logger in every action.
- Steps to Create Custom Middleware
- We will create a separate class for the implementation of custom middleware.
- .NET core provides a middleware class to create custom middleware, or you can create a normal class.
- In our demo we will use Middleware class for implementation.
- First, we created a folder for middleware in our project for maintain the project structure.
- Right click on that folder > Add > New Item as per below.
It will open new modal for item or template selection, select Middleware Class and add.
Once you add, it will create a class with below code:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using System.Threading.Tasks;
namespace MiddlewareChecking.Middleware
{
// You may need to install the Microsoft.AspNetCore.Http.Abstractions package into your project
public class RequestLogMiddleware
{
private readonly RequestDelegate _next;
public RequestLogMiddleware(RequestDelegate next)
{
_next = next;
}
public Task Invoke(HttpContext httpContext)
{
return _next(httpContext);
}
}
// Extension method used to add the middleware to the HTTP request pipeline.
public static class RequestLogMiddlewareExtensions
{
public static IApplicationBuilder UseRequestLogMiddleware(this IApplicationBuilder builder)
{
return builder.UseMiddleware<RequestLogMiddleware>();
}
}
}
In this demo, I am creating custom middleware for logging requests. The updated code includes implementation to log only request methods, excluding CSS, image, and JS files.
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using System.Threading.Tasks;
namespace MiddlewareChecking.Middleware
{
public class RequestLogMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<RequestLogMiddleware> _logger;
public RequestLogMiddleware(RequestDelegate next, ILogger<RequestLogMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task Invoke (HttpContext httpContext)
{
if (!IsStaticFileRequest(httpContext.Request.Path))
{
_logger.LogInformation("Request received: {Method} {Path}", httpContext.Request.Method, httpContext.Request.Path);
await _next(httpContext);
_logger.LogInformation("Response sent: {StatusCode}", httpContext.Response.StatusCode);
}
}
private bool IsStaticFileRequest(PathString path)
{
string[] staticFileExtensions = { ".css", ".js", ".jpg", ".jpeg", ".png", ".gif", ".ico" };
string pathString = path.Value.ToLower();
foreach (var extension in staticFileExtensions)
{
if (pathString.EndsWith(extension))
{
return true;
}
}
return false;
}
}
// Extension method used to add the middleware to the HTTP request pipeline.
public static class RequestLogMiddlewareExtensions
{
public static IApplicationBuilder UseRequestLogMiddleware(this IApplicationBuilder builder)
{
return builder.UseMiddleware<RequestLogMiddleware>();
}
}
}
Above is the code for the custom middleware, you can create any type of custom middleware like Exception Handling, Session middleware as per requirement.
- Use custom middleware in the HTTP pipeline
- We can use this custom middleware in the HTTP pipeline in Startup.cs file. Like below,
Conclusion
ASP.NET Core Middleware is a powerful feature that enables developers to manage HTTP requests and responses in a correct order. By creating custom Middleware components, we can add custom logic as per requirement, modify requests, and process responses to making web application work just the way they want them to.
After reading this post, if you're looking to experience the potential of ASP.NET Core Middleware, our team of skilled .NET developers specializes in crafting custom Middleware components tailored to your specific needs. So, hire .NET developers from The One Technologies and streamline your HTTP request handling, enhance user experience, and optimize performance.
More examples can be found at this link: -
https://blog.stackademic.com/how-to-create-and-use-the-custom-middleware-in-net-core-8516e9884abf
About Author
Pratiksha Pansara is a seasoned Sr. .NET Developer with a passion for continuous learning and growth. Since May 2019, she has been on a journey of self-improvement, constantly honing her skills and expanding her knowledge base to better serve her clients. With a keen interest in the .NET framework and related technologies, Pratiksha aspires to elevate her expertise and delve into the world of Angular development. Armed with a wealth of experience in .NET, she is poised to lead impactful projects and drive innovation in the field.