Minimal API in .NET 8
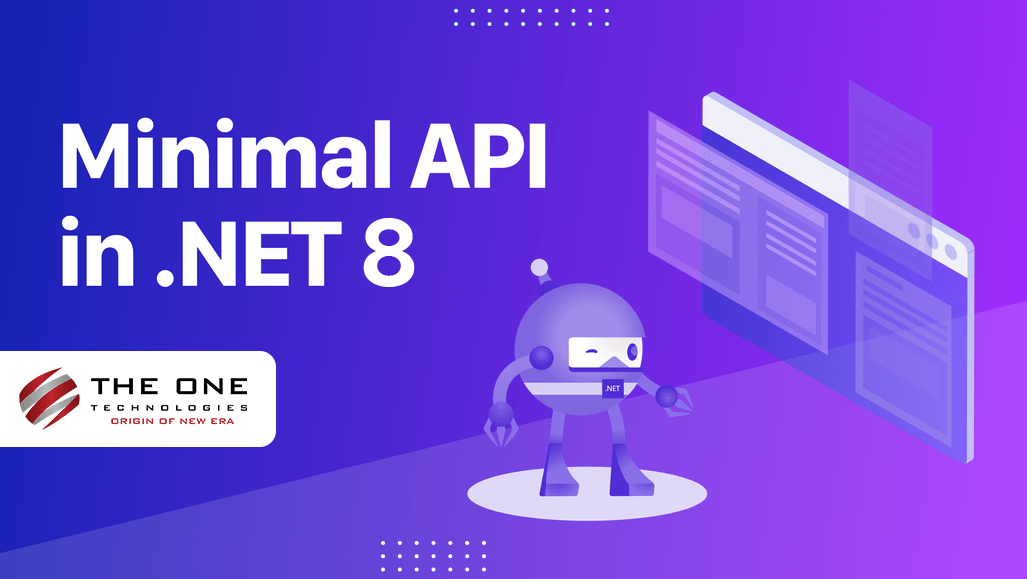
Introduction
Minimal APIs are designed to streamline the development process by reducing boilerplate code and configuration, allowing developers to focus more on writing business logic. With .NET 8, you can create APIs with just a few lines of code, making it easier to iterate and maintain your applications. Also, it offers improved performance and scalability, making it an excellent choice for modern web development projects. Get started with Minimal APIs today and experience the simplicity and efficiency they bring to your ASP.NET Core applications!
Table of Content
- Key Points
- Steps for Creating a Minimal API in .NET Core
- Advantages of Minimal APIs in .NET 8
- Limitations of Minimal APIs in .NET 8
- Conclusion
Key Points
Streamlined Development:
- Minimal APIs allow developers to define endpoints with minimal code, reducing the boilerplate typically associated with setting up a web service.
- This streamlined approach accelerates the development process, enabling quicker iterations and faster time-to-market.
Enhanced Performance:
- By minimizing abstractions and middleware, Minimal APIs offer improved performance.
- Direct handling of HTTP requests leads to faster execution times and reduced latency.
Ease of Learning:
- The simplified syntax and reduced complexity make Minimal APIs an excellent entry point for new developers.
- Experienced developers can also benefit from the ease of use, allowing them to focus on core logic rather than configuration.
Flexibility and Extensibility:
- Minimal APIs provide the flexibility to integrate only the necessary features, avoiding the overhead of unnecessary components.
- Developers can easily extend functionalities as needed, tailoring the API to specific requirements.
Modern Features:
- .NET 8 introduces new capabilities such as improved async/await support, enhanced route handling, and robust dependency injection.
- These features empower developers to build more responsive and maintainable applications.
Scalability:
- Despite their minimalistic nature, Minimal APIs are scalable and capable of supporting large, complex applications.
- They are particularly well-suited for microservices architectures, where each service can be developed, deployed, and scaled independently.
Ideal for Specific Use Cases:
- Minimal APIs excel in scenarios where lightweight, efficient endpoints are required, such as IoT applications, mobile backends, and serverless functions.
- Their low overhead and rapid startup times make them perfect for environments with constrained resources.
Improved Testing and Debugging:
- The simplicity of Minimal APIs facilitates easier testing and debugging.
- Developers can quickly set up and run unit tests, ensuring the reliability and robustness of their APIs.
Community and Ecosystem Support:
- As part of the ASP.NET Core ecosystem, Minimal APIs benefit from a rich set of libraries, tools, and community support.
- Extensive documentation and examples are available to help developers get started and overcome common challenges.
Steps for creating a Minimal API in .NET core
There are some easy steps that need to be followed.
- Start Visual Studio 2022 and select Create a new project.
- Select the ASP.NET Core Web API template and select Next
While creating a new Project make sure you don't check the checkbox for use controller.
After creating the project, we can see that there is only program.cs file, no controller.
- We must write all the code in program.cs file.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
app.UseSwagger();
app.UseSwaggerUI();
// Middleware to handle JSON response
app.Use(async (context, next) =>
{
context.Response.Headers.Add("Content-Type", "application/json");
await next();
});
// GET all items
app.MapGet("/Comapanytodos", () =>
{
return CompanyData.Items;
});
// GET single item by ID
app.MapGet("/Comapanytodos/{id}", (HttpContext context, int id) =>
{
var company = CompanyData.Items.FirstOrDefault(t => t.Id == id);
if (company != null)
return Results.Ok(company);
else
return Results.NotFound();
});
// POST a new item
app.MapPost("/Comapanytodos", (HttpContext context) =>
{
var company = context.Request.ReadFromJsonAsync<CompanyItem>().Result;
if (company != null)
{
company.Id = CompanyData.Items.Count > 0 ? CompanyData.Items.Max(t => t.Id) + 1 : 1; // Generate new ID
CompanyData.Items.Add(company);
return Results.Created($"/Comapanytodos/{company.Id}", company);
}
else
{
return Results.BadRequest("Invalid todo item");
}
});
// PUT update an item
app.MapPut("/Comapanytodos/{id}", (HttpContext context, int id) =>
{
var company = CompanyData.Items.FirstOrDefault(t => t.Id == id);
if (company != null)
{
var updatedCompany = context.Request.ReadFromJsonAsync<CompanyItem>().Result;
if (updatedCompany != null)
{
company.Technology = updatedCompany.Technology;
company.Vacancy = updatedCompany.Vacancy;
return Results.Ok(company);
}
else
{
return Results.BadRequest("Invalid todo item");
}
}
else
{
return Results.NotFound();
}
});
// DELETE a item
app.MapDelete("/Comapanytodos/{id}", (int id) =>
{
var company = CompanyData.Items.FirstOrDefault(t => t.Id == id);
if (company != null)
{
CompanyData.Items.Remove(company);
return Results.Ok();
}
else
{
return Results.NotFound();
}
});
app.Run();
Model:-
public class CompanyItem
{
public int Id { get; set; }
public string Technology {get; set; }
public string Vacancy { get; set; }
}
// In-memory data storage
public static class CompanyData
{
public static List<CompanyItem> Items { get; } = new List<CompanyItem>
{
new CompanyItem { Id = 1, Technology = "Laravel", Vacancy = "No" },
new CompanyItem { Id = 2, Technology = "Android", Vacancy = "Yes" },
new CompanyItem { Id = 3, Technology = "Dot Net", Vacancy = "Yes" }
};
}
- GET, POST, PUT and DETETE
I've mentioned all the code above for your reference. Used Static data.
Advantages of Minimal APIs in .NET 8
Simplicity and Ease of Use
- Minimal APIs help reduce boilerplate code, making it quicker and simpler to set up web services. Their concise syntax is less intimidating for new developers, which speeds up the development process.
Performance
- Fewer layers of abstraction result in better performance compared to traditional controller-based approaches.
- Direct handling of HTTP requests can lead to more efficient processing.
Flexibility
- Developers can integrate necessary features without adhering to a rigid framework structure.
- This flexibility allows for customized and optimized application development.
Modern Features
- .NET 8 introduces improved async/await integration, advanced route handling, and robust dependency injection, enhancing the capabilities of Minimal APIs.
- These enhancements make Minimal APIs more powerful and versatile.
Scalability
- Despite their minimal setup, Minimal APIs can support large, production-grade applications.
- They are suitable for microservices, enabling each service to be developed, deployed, and scaled independently.
Ideal for Specific Use Cases
- Particularly well-suited for microservices, serverless functions, and rapid prototyping.
- Low overhead and quick startup times are beneficial for serverless computing.
Limitations of Minimal APIs in .NET 8
Limited Built-in Features
- Minimal APIs do not come with the extensive built-in features of traditional ASP.NET Core MVC, such as model binding, validation, and filters.
- Developers may need to implement these features manually or through additional libraries.
Learning Curve
- While the syntax is simpler, understanding how to extend Minimal APIs to match the functionality of traditional frameworks can require additional learning and experimentation.
Less Mature Ecosystem
- As a newer approach, Minimal APIs may have a less mature ecosystem compared to the well-established ASP.NET Core MVC.
- There may be fewer community resources, tutorials, and third-party integrations available.
Suitability for Complex Applications
- For very complex applications with extensive requirements for data handling, validation, and intricate routing, the traditional MVC approach might be more suitable.
- Minimal APIs may not provide the necessary structure and built-in features for such applications.
Maintenance and Readability
- As the application grows, maintaining many endpoint definitions in a minimal style can become cumbersome and less readable compared to a more structured MVC approach.
Dependency Injection and Middleware Limitations
- While Minimal APIs support dependency injection and middleware, the approach to these can be less intuitive and more limited compared to the traditional MVC setup.
Conclusion
Minimal APIs in .NET 8 provide a streamlined approach for developers aiming to create efficient and scalable web services. These APIs emphasize simplicity, high performance, and flexibility, making them ideal for microservices, lightweight applications, and quick prototyping. However, developers should be mindful of their limitations, such as the necessity for manual implementation of certain features and potential difficulties in managing more complex applications. By comprehending both the benefits and drawbacks, developers can better decide when and how to effectively use Minimal APIs.
Are you planning to leverage the power of Minimal APIs in .NET 8 for your projects? Our .NET development company specializes in harnessing the potential of cutting-edge technologies like Minimal APIs to deliver efficient and scalable web services. Connect with us and see how our dedicated development team can help you navigate the complexities and maximize the benefits of Minimal API development.
Author Bio
Yash Dubey is a Junior .NET Developer at The One Technologies, embarking on his journey in the IT industry since December 2021. Starting as a trainee, Yash has progressed steadily to his current role, overcoming task difficulties and honing his skills to work independently. Beyond coding, his interests span cricket, chess, and badminton. Looking ahead, his future professional goal is to delve into the realms of Artificial Intelligence and Machine Learning alongside his passion for .NET development.