7 Best Practices to Boost React Native App Performance
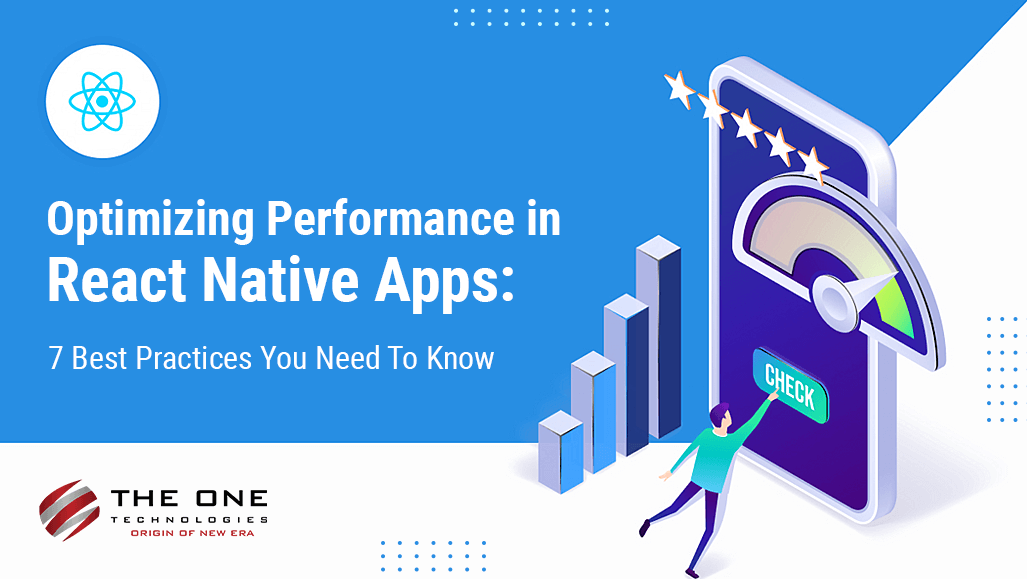
Table of Content
- Introduction
- Key Factors Affecting React Native Performance
- 7 Best Practices for Optimizing React Native Performance
- Why Optimizing Performance of React Native apps is Important?
- Wrapping Up
- People Also Ask
Introduction
With the mobile app demand ongoing, developers have been working diligently to provide fast performance to end-users on multiple platforms. With its cross-platform nature and recognizable JavaScript syntax. React Native has become a widely used option for developing mobile applications with ease. Optimal performance in React Native applications, though, comes with a set of challenges. These are different from others and need to be overcome to provide seamless and responsive user interactions.
Let's dive into the nitty-gritty of performance optimization in React Native applications. And learn the top practices that every developer should know. Grasping the root causes will enable developers to effortlessly accelerate their React Native apps. Be you a React Native development agency or a solo developer, learning these practices is crucial to provide world-class mobile experiences. So, let's dive in!
Key Factors Affecting React Native Performance
1. Memory Management
To avoid memory leaks and improve overall app performance, React Native requires effective memory management. Using tools like React Native Debugger or Chrome Developer Tools to identify memory snapshots, developers should be watchful in spotting and fixing memory leaks. Improved performance of apps and a large reduction in memory cost can also be achieved by implementing effective data structures and enhancing picture loading and caching.
2. JavaScript Execution
JavaScript execution has a direct impact on React Native application responsiveness. Developers should reduce excessive work in render routines to improve efficiency because large computations might cause UI jank. Long-running operations must be performed by employing asynchronous programming strategies. Such as Promises and async/await so that they don't block the main thread. React Developer Tools and other profiling tools help determine and enhance critical code paths for improved performance.
3. Rendering
Smooth user experiences in React Native apps are mostly dependent on rendering efficiency. Developers can reduce needless re-renders using methods like shouldComponentUpdate or React.memo to improve rendering performance. Also, you may increase rendering speed and user interface responsiveness by refining component structure and reducing the number of costly operations used in render functions.
4. Network Requests
React Native app speed depends heavily on effective network request management, particularly in situations where frequent data fetching or changes are required. To reduce network overhead and latency, developers should put techniques like request batching, caching, and API endpoint optimization into practice. While methods like delayed loading can help optimize initial data loading for better app performance, libraries like Axios or Fetch API offer functionality for effective network request management.
5. Code Optimization
Optimizing the efficiency and code organization of React Native applications is necessary in order to enhance their performance. Through the elimination of unused dependencies, the use of code-splitting practices. And the application of tree shaking, developers can focus on reducing the bundle size. To further guarantee that the app functions smoothly and effectively across various platforms and devices, bottlenecks can be found and addressed with the aid of performance monitoring tools and routine code reviews.
7 Best Practices for Optimizing React Native Performance
1. Effective State Data Handling
Poor state management can lead to inconsistent data, which might lead to unpredictable behavior that would be difficult to locate and solve. Not storing extraneous data within the state. Which could decrease the speed of the application and complicate debugging, is one of the integral parts of effective state management. Make sure that every state you store is required for the component to function.
Using immutability strategies such as the Object.assign() method or the spread operator is another efficient way to update the state.
Have a look at the example given below:
import React, { useState } from "react";
function MyComponent() {
const [state, setState] = useState({
count: 0,
text: "Hello",
});
function handleClick() {
setState((prevState) => {
return Object.assign({}, prevState, { count: prevState.count + 1 });
});
}
return (
<div>
<button onClick={handleClick}>Increment Count</button>
</div>
);
}
In this instance, the state is updated by the handleClick method using the setState hook. Nevertheless, it duplicates the prior state with the count field changed and creates a new object using the Object.assign() method rather than altering the state object directly. By using this method, React's virtual DOM may detect that you have altered the state and re-render the component.
2. Remove Console Statements
One of the most popular methods for debugging JavaScript applications in general, including React Native apps, is to use console.log statements. When publishing React Native apps, leaving the console statements in the source code can result in significant JavaScript thread bottlenecks.
3. Use Virtualized Lists
Since virtualized lists effectively show only the items visible on the screen, FlatList and SectionList are excellent choices for displaying more items than the standard ListView. This maximizes rendering speed and memory utilization, particularly for large lists.
import React from 'react';
import { FlatList, Text } from 'react-native';
const data = [...]; // An array of data
const VirtualizedListExample = () => {
return (
<FlatList
data={data}
keyExtractor={(item) => item.id}
renderItem={({ item }) => <Text>{item.title}</Text>}
/>
);
}
Also, VirtualizedLists provide particular props for fine-grained control over optimization configuration, like updateCellsBatchingPeriod to set the ms interval between batch renders and maxToRenderPerBatch to control the number of items rendered per batch when scrolling through the list. You may improve it even further by using FlashLists, which provide a set of additional props to further adjust performance and render stuff quickly.
4. Minimizing Re-renders
React Native app performance can be optimized by minimizing re-renders. Excessive re-rendering of a component may lead to wasting resources and non-responsive apps. Applying shouldComponentUpdate or using React.memo, which gives devs the ability to decide when exactly a component would re-render when props or state changes, is one effective way to minimize re-renders. By thorough specification of when and under what conditions a component updates, devs can prevent unnecessary re-renders and streamline the overall efficiency of their app.
All things considered, reducing re-renders is a crucial recommended practice for maximizing React Native speed. Developers can make sure that their apps stay responsive, quick, and effective even as they get more complicated by properly controlling component updates and streamlining component architecture.
5. Integrate Suitable Navigation
While most apps now offer a smooth experience, there are still a few navigation-related difficulties that need to be resolved. The React Native team has been working on these issues for some time. Difficult navigation between screens can prevent users from using your app at all.
6. Optimize Your Images
Images frequently affect performance and increase the size of apps. Take into consideration the following methods:
- Utilize appropriate picture formats (like JPEG and PNG) and reduce the size of your photos by compressing them.
- Use utilities like react-native-fast-image or react-native-image-resizer to optimize image loading and caching.
- Lazy-load images to reduce needless network queries and speed up app load times.
7. Improve Debugging Speed
The debugging rate greatly impacts productivity and app speed. You'll see that an app's agility might be negatively impacted by its bug count. Flipper is the best option if you want to expedite the procedure and guarantee that all bugs are eliminated. Both native app platforms and React Native support it effectively.
The design includes a thorough record and a network inspector. By incorporating it into your code and keeping an eye on the methods, logic, and functions, you may reduce the amount of time you spend debugging.
Why Optimizing Performance of React Native Apps Important?
Improving React Native app efficiency is essential for many compelling reasons. First, user demands for highly responsive and seamless experiences have never been higher. So anything short of fast screen loading, responsive UI, and responsive interactions can quickly lead to user frustration. These can actually culminate in lower user engagement and retention. Through performance tuning, developers are able to make sure that their React Native applications are offering a seamless and wonderful user experience. Hence, this improves user satisfaction and generating positive app reviews and ratings.
The effect of performance optimization carries over to business results. Research time and again demonstrates that quicker apps have improved retention rates and better conversion rates. Enhanced performance not just increases user interactions and satisfaction but also leads to enhanced conversions, revenue, and company reputation. Moreover, by minimizing server load, bandwidth utilization, and infrastructure needs. Optimized performance can help decrease operation costs.
Wrapping Up
Mastery of optimizing performance in React Native applications is key to the delivery of best-in-class user experiences and driving business success. By adopting the 7 best practices presented herein, developers are able to easily improve the responsiveness. And efficiency of their app significantly. Furthermore, the use of profiling tools, ongoing testing, and constant optimization activities are necessary for effective detection. And fixing of performance bottlenecks. With dedication to excellence in performance optimization, developers will be able to take their React Native apps to unprecedented levels. Satisfying users and becoming the epitome of long-term success.
If you have already got a plan to develop a React Native application and are in search of a trusted service provider for your project, then your search ends here. Get in touch with The One Technologies and see how our proficiency as one of the best React Native development companies in USA. We can revolutionize the performance of your app and take your business to new heights.
People Also Ask
Q1. What are the main performance bottlenecks in React Native apps?
Performance bottlenecks in React Native applications are often caused by memory management, JavaScript execution, rendering efficiency, network queries, and code optimization. These problems need to be identified and resolved in order to enhance app performance.
Q2. What types of React Native apps can your team develop?
Our staff is proficient in creating a wide range of React Native applications, including those for productivity, entertainment, social media, eCommerce, and more. We can adapt solutions to your particular business demands thanks to our experience.
Q3. Can you integrate third-party APIs and services into React Native apps?
Yes, we are skilled in incorporating a variety of third-party APIs and services into React Native apps, including payment gateways, social media connectors, analytics tools, and more. Our developers are adept in incorporating APIs to enhance the features and capabilities of your app.
Q4. How can I enhance the rendering efficiency in my React Native app?
Optimize component structure, use less expensive operations in render methods, and reduce the number of unnecessary re-renders by utilizing techniques like shouldComponentUpdate or React.memo.
Q5. Do you offer performance optimization services for existing React Native apps?
Yes, we provide performance optimization services for existing React Native applications. Our staff can evaluate your app's functioning, identify any bottlenecks, and implement best practices to improve responsiveness, performance, and overall user experience.