SignalR in .NET Core: Enabling Real-Time Web Applications
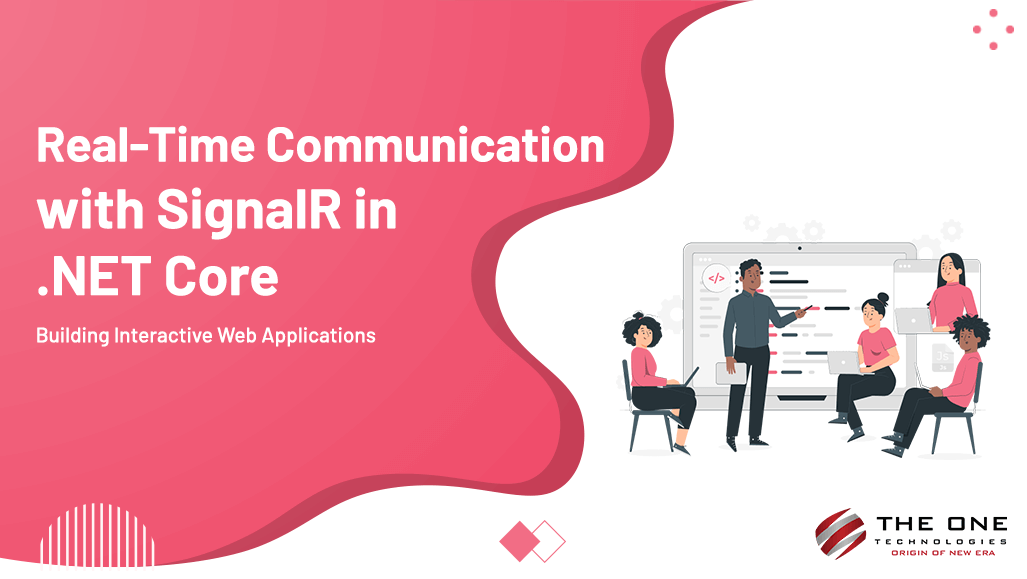
SignalR is a bi-directional communication library that makes use of the .NET framework to facilitate real-time applications, where the client and server have a constant connection and can exchange information. An example where this may be beneficial is in the development of online multiplayer games, chat applications, or real-time data displays where updates need to be delivered instantaneously.
Start an exhilarating journey into real-time communication with SignalR in .NET Core! As a .NET Core developer, you'll discover the power of building interactive web applications that engage users like never before.
Table of Contents
- Step-by-Step Guide to SignalR Integration
- SignalR with two projects
- Conclusion
- Demo projects can be found at this link
Step-by-Step Guide to SignalR Integration
1. Start a fresh project with .NET Core.
2. Set up the NuGet package SignalR.
To your project, add the SignalR package: SignalR by Microsoft.AspNetCore
3. Establish the SignalR Hub
A SignalR hub is a class that manages client-server communication by acting as a high-level pipeline. For your hub, create a new class:
using Microsoft.AspNetCore.SignalR;
using System.Threading.Tasks;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string msg)
{
await Clients.All.SendAsync("ReceiveMessage", user, msg);
}
}
The hub in this example contains a SendMessage method that sends out a message to every client that is connected.
4. Setup SignalR using Startup.cs.
The ConfigureServices and Configure methods included in the Startup.cs file should be used to configure SignalR:
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
}
public void Configure(IApplicationBuilder app)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ChatHub>("/chatHub");
endpoints.MapDefaultControllerRoute();
});
}
}
5. Establish a connection from the client
The SignalR client library is available for usage on the client side (in JavaScript within a web application). As an illustration, using JavaScript:
<!-- Add the SignalR library here -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/microsoft-signalr/3.1.10/signalr.min.js"></script>
<script>
const connection = new signalR.HubConnectionBuilder().withUrl("/chatHub").build();
connection.start().then(function () {
connection.invoke("SendMessage", "Neha", "Hello, Nisha!");
}).catch(function (err) {
console.error(err.toString());
});
connection.on("ReceiveMessage", function (user, msg) {
console.log(user + " says: " + msg);
});
</script>
6. SignalR call from Action
You may use dependency injection to inject an instance of the SignalR hub context into your controller or service so that you can call SignalR from an action in a.NET Core application.
Following is a detailed how-to:
Assuming you have a SignalR hub as shown in the example above (eg, ChatHub), let's create a controller that calls SignalR from an action:
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.SignalR;
using System.Threading.Tasks;
public class MyController : Controller
{
private readonly IHubContext<ChatHub> _hubContext;
public MyController(IHubContext<ChatHub> hubContext)
{
_hubContext = hubContext;
}
public IActionResult Index()
{
return View();
}
[HttpPost]
public async Task<IActionResult> SendMessage(string user, string msg)
{
// Your business logic here...
// Send the message to all connected clients
await _hubContext.Clients.All.SendAsync("ReceiveMessage", user, msg);
return Ok();
}
}
The SendMessage action in this example is designated to handle HTTP POST requests via the [HttpPost] attribute. The action calls, after your business logic (replace the remark with your actual code). it calls _hubContext.Clients.All.SendAsync("ReceiveMessage", client, msg); to broadcast a message via the ChatHub to every client that is connected.
Verify that the SignalR hub and MyController are being used by your Startup.cs file:
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
services.AddControllersWithViews();
}
public void Configure(IApplicationBuilder app)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ChatHub>("/chatHub");
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
You can have a form in your client-side JavaScript code that makes a POST request to the SendMessage action:
<form id="messageForm">
<input type="text" id="user" placeholder="User" />
<input type="text" id="message" placeholder="Message" />
<button type="button" onclick="sendMessage()">Send Message</button>
</form>
<script>
function sendMessage() {
var user = document.getElementById("user").value;
var msg= document.getElementById("message").value;
fetch('/My/SendMessage', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ user: user, message: msg}),
});
}
</script>
7. Launch the application.
You should be able to observe the SignalR hub in operation when you run your.NET Core application.
This is a rudimentary configuration; SignalR offers other tools to control authorization, connections, and other aspects. Check the official documentation for advanced usage and more detailed information.
SignalR with two projects
One project must be set up as the hub (server), and the second project has to connect to and communicate with the hub (client) in order to use SignalR with two projects. These kinds of scenarios, in which numerous components exchange real-time information with one another, are common in distributed systems and microservices designs.
Using two projects—a server project that houses a SignalR hub and a client project that connects to the hub—this is a simple lesson on configuring SignalR.
Server Project (SignalR Hub):
1. Install SignalR NuGet Package:
Add the SignalR package to your server project: Microsoft.AspNetCore.SignalR
2. Create a SignalR Hub:
Make a new.NET Core project and include the SignalR hub class (the same one as in the earlier examples).
// ServerProject/ChatHub.cs
using Microsoft.AspNetCore.SignalR;
using System.Threading.Tasks;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string msg)
{
await Clients.All.SendAsync("ReceiveMessage", user, msg);
}
}
3. Configure SignalR in the file Startup.cs:
Configure SignalR in the Startup.cs file of the server project.
// ServerProject/Startup.cs
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
}
public void Configure(IApplicationBuilder app)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ChatHub>("/chatHub");
// Other endpoint mappings...
});
}
}
Client Project:
1. Add the SignalR client package to the client project.
Microsoft.AspNetCore.SignalR.Clientotne
Link to the SignalR hub and handle events in your client code (such as a console application, Blazor app, or other web application).
// ClientProject/Program.cs (Console Application example)
using System;
using Microsoft.AspNetCore.SignalR.Client;
class Program
{
static async Task Main(string[] args)
{
var connection = new HubConnectionBuilder()
.WithUrl("http://localhost:5000/chatHub") // Replace with your server URL
.Build();
connection.On<string, string>("ReceiveMessage", (user, msg) =>
{
Console.WriteLine($"{user} says: {msg}");
});
await connection.StartAsync();
// Perform other tasks or await client input...
await connection.StopAsync();
}
}
Adjust the WithUrl URL to correspond with the SignalR hub's URL.
2. Include the SignalR client library.
When prompted to add a client-side library:
Select unpkg for Provider
For Library, type @microsoft/signalr@latest .
Select Choose specific files you want to work with, expand the dist/browser folder and choose signalr.js and signalr.min.js.
Put wwwroot/js/signalr/ as the Target Location.
Click Install.
3. Execute the Both Projects:
Run the SignalR hub server project.
Run the project for the client.
You can try sending messages from the hub to the client and vice versa once communication has been established between the SignalR hub and the client.
Although this is a simple example, depending on your application's requirements, you may also need to take security, error handling, and other issues into account in a real-world setting.
Conclusion
This blog post demonstrates how SignalR is a tool that can recognize the steps involved in developing real-time online functionality. It makes it simpler for developers to manage client-server relationships and push content updates to the client side from the SignalR Hub because it includes an ASP.NET Site server library and a Javascript client library. In order to use this service for web apps and have real-time data and functionality, developers must first install the SignalR library to an existing ASP.NET application. The SignalR service assists.NET development firms in building reliable, fast, and scalable web applications.
After reading this post, you must want to experience the power of SignalR to propel your web applications into the realm of real-time functionality and engagement. So, what are you waiting for? Hire a trustworthy .Net development company and take your projects to the next level.
Demo projects can be found at this link
Author Bio
Nutan is a seasoned Sr .NET Developer at The One Technologies having over 3 years of experience in IT industry. Since commencing her journey in 2019, she has shown remarkable career progression driven by a passion for learning and innovation. With expertise in C# programming and the .NET Framework, she excels in ASP.NET for web development, crafting dynamic and interactive applications. She aims to become a recognized expert in .NET development, contributing to impactful projects and assuming leadership roles.