Useful and Handy Commands for Development
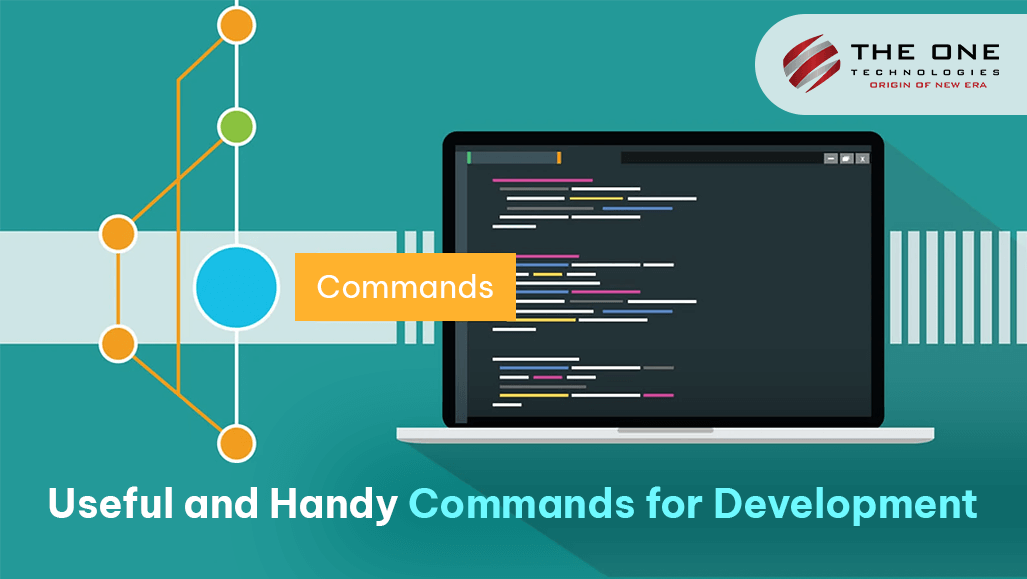
Introduction
We use GUI (Graphical User Interface) in our respective Operating Systems. But, the CLI (Command Line Interface) is still fast, reliable, and widely used by professionals.
The most common advantage of CLI is that we can do everything from a single terminal, but for GUI, we might need a new window for each task.
So, below are some commands of different areas that can be helpful in your day-to-day life.
There shall be some commands you may or may not be aware of. But knowing that we have shortcuts like these can be a lifesaver sometimes.
Table Of Contents
- Prerequisite for commands
- GIT Commands
- Node JS Framework Commands
- .Net Framework Commands
- Windows OS Commands
- PRO TIP
- Conclusion
Prerequisite for commands
All the commands related to files or folders are needed to execute in the target folders only. For that, you shall open the terminal from the target folder or open it from anywhere and navigate to the folder.
GIT
Git is one of the source control widely used by developers. We use it for its efficient branch system.
So, here are some commands related to git to delete the deleted branches from your local repo. Kindly note that you might want to use git bash to use this command if you are using Windows.
Common Commands
Here are below some general git commands that you need to use daily.
Commands:
Terminal: Bash for all
Repository:
# Init: Initialize a new repository.
git init
# Clone: To clone a new repository.
git clone <Remote-URL>
# Remote: To list all named repositories.
git remote -v
# Pull: To take a pull from named remote.
git pull <Branch-name> <Remote-URL/Remote-name>
# Push: To send commits to the remote repository.
git push <Remote-URL/Remote-name> <Branch>
# Config: To alter a configuration of the git.
git config <Setting> <Command>
Example:
git config user.email "<email-address>"
git config user.name "<user-name>"
Files and Commits:
# Add: To stage all un-staged modified files.
git add .
# Commit: To add a commit locally with a message.
git commit -m "<Commit-message-in-quotes>"
# Status: To get the current state of the repository.
git status
# Stash: To store the current changes locally with untracked(new) files.
git stash save "<Stash-Nessage>"
Branches:
# Create a new branch
$ git branch <Branch-Name>
# List all remote or local branches of git
$ git branch -a
# Delete a branch
$ git branch -d <Branch-Name>
# Checkout:
# Checkout an existing branch
$ git checkout <Branch-Name>
# Checkout and create a new branch with that name
$ git checkout -b <New-Branch-Name>
# Merge: To merge the mentioned branch changes into the current branch.
git merge <Branch-Name>
# Note: We need to manually resolve the found conflicts from an IDE as we need to manually review and confirm which piece of code we need to keep.
# Rebase: To merge the mentioned branch changes into the current branch but, without the commit history as if the branch is recently checked out.
git branch <Branch-Name>
Tags:
# List tags
git tag
# List tags with search
git tag -l "<Search-Token>"
# Create a tag
git tag -a <Tag-Name> HEAD -m "<Tag-Message>"
# Sync changes with Remote and specific tag
git push <Remote-Name> <Tag-Name>
# Note: To add tag for a specific commit, we need to add tag name in the push as above.
Reference Link: http://guides.beanstalkapp.com/version-control/common-git-commands.html
If you are searching for some commands for some specific situations, you can find them below.
Remove all deleted Remote Git Branches.
When we fetch, pull, or checkout a branch from a remote, a remote copy gets saved in our system, and it does not delete automatically from your system after it gets deleted from the remote. We can do it by the below command.
Command:
# Terminal: Bash
git fetch --prune
Remove all deleted Remote Git Branches checked out locally.
The above command will only remove the remote branches from your remote local, but if you have checked out a git branch in local and it gets deleted from the remote, it will still stay in as your local branch. To delete those local branches, you can use the below command.
Command:
# Terminal: Bash
git fetch -p ; git branch -r | awk '{print $1}' | egrep -v -f /dev/fd/0 <(git branch -vv | grep origin) | awk '{print $1}' | xargs git branch -d
Reset files from the most recent commit before a push.
There are times when we take a pull of a branch into the current git branch and want to revert it just after that, or the wrong git branch got pulled. So to revert the changes and roll back the changes, we can use the below command.
Command:
# Terminal: Bash
git reset --hard
Node JS Framework
Node JS is one of the fastest growing javascript frameworks in developing websites and applications. Despite being fast and reliable, we might need manual interference to fix issues while working with it. One of them is listed below.
Delete all node_modules folders from all subfolders from the current directory.
When we work in NodeJs applications, we can come across microservice/micro-frontend applications. They tend to have multiple node_modules folders inside the root directory. If the folder structure is not standard, we might see more than one node_modules folder in that project.
Hence while working on these projects, we might need to remove all the dependencies by deleting the node_modules folder(s). So, rather than searching for each instance of the folder and deleting it, we can use the below command.
Command:
# Terminal: Any
# One time install of package.
npm install rimraf -g
# Command to remove all node_modules folders recursively from current directory.
rimraf .\**\node_modules
.Net Framework
.NET is a software development framework created by Microsoft that provides a platform for building Windows desktop applications, web applications, and services using various programming languages such as C#, Visual Basic, and F#. It includes a runtime environment, a class library, and development tools for application creation, deployment, and management.
Delete all bin and obj folders from the current directory
In .Net projects, we can expect a multi-tier application where logic and code get divided among different apps and libraries. Hence, each app/library has its own bin and obj folder. We don't have any issues with that, but manually deleting the bin and obj folders can be tedious if needed. So, we can use the below command. It will search for all the bin and obj folders of the solution and delete them on a single execution.
Command:
# Terminal: CMD, Powershell
Get-ChildItem .\ -include bin,obj -Recurse | foreach ($_) { remove-item $_.fullname -Force -Recurse }
Windows OS
Windows is one of the widely used Operating Systems. But despite its rich mouse-friendly UI, performing some tasks can be tedious, long, and boring. Moreover, you might need some direct commands, which can save you some seconds.
Robocopy to copy/move files
Robocopy is an inbuilt tool of Windows that is only usable from the terminal for now. It is a fast and reliable way to copy/move files from one location to another.
Many discussions are floating on the internet about why this is not used natively for copying/moving files in the code. Some 3rd parties applications run Robocopy internally, like TeraCopy, etc. You can use the below command if you don't want to install any other software and still want faster copy/move speeds.
Command:
# Terminal: CMD, Powershell
robocopy "<Source Path>" "<Destination Path>" /move /is /e /compress /mt:128
To move the files and folders using Robocopy, append move to the above command. Also, if you want to create a new root folder for the destination, add the name to the destination path.
Add ports to the Windows Firewall
In corporate places where security is our utmost priority, we tend to disable all the ports for the public by default. So we need to make the ports public if we host a website or start an application that runs on an available port. To achieve this, we need to enable the port in the firewall for inbound and outbound. When the following commands execute in the terminal with elevated privileges, they create an entry in the inbound and outbound rules.
Commands:
Inbound:
# Terminal: CMD, Powershell
New-NetFirewallRule -DisplayName '<Port Number>' -Profile 'Domain,Private,Public' -Direction Inbound -Action Allow -Protocol TCP -LocalPort <Label>
Outbound:
# Terminal: CMD, Powershell
New-NetFirewallRule -DisplayName '<Port Number>' -Profile 'Domain,Private,Public' -Direction Outbound -Action Allow -Protocol TCP -LocalPort <Label>
As per the above commands, we have labeled the rule as the port number for easy searching. You can use the name of your choice.
Remove all blank folders from a given path
If we are working in a console/desktop application, the code can create blank folders during execution if the code is in that aspect. We need to clean up the empty folders by deleting them. We are also considering the folders that contain other subfolders that either have empty subfolders or nothing. Here, the default command of folder deletion will not work as the folder has something inside it, although it has an empty subfolder(s). So, the below command can find these subfolders and delete them recursively.
Command:
# Terminal: Powershell
for /f "delims=" %d in ('dir /s /b /ad ^| sort /r') do rd "%d"
Pro Tip
Generally, the commands work in a single type of terminal, and it would be great if we confirm that before executing.
I know only the commands above that can be generic and helpful in daily tasks. If you know any such terminal commands and want to add them here, kindly let me know. I will love to add them to this blog with your mention.
Conclusion
There are many commands available that can make our life easier. They shall not replace the GUI we are using in all OS, but they can shorten the duration of the tasks where we use them.
About Author
Rahul Mahadik started his career journey in 2015 in a .net development company. Currently, he is a full-stack team leader at The One Technologies.
He loves to solve complex issues while taking care of modernizing and optimizing. He is fond of listening to music, spending time with family & friends, and watching movies.