What's new in .NET 7
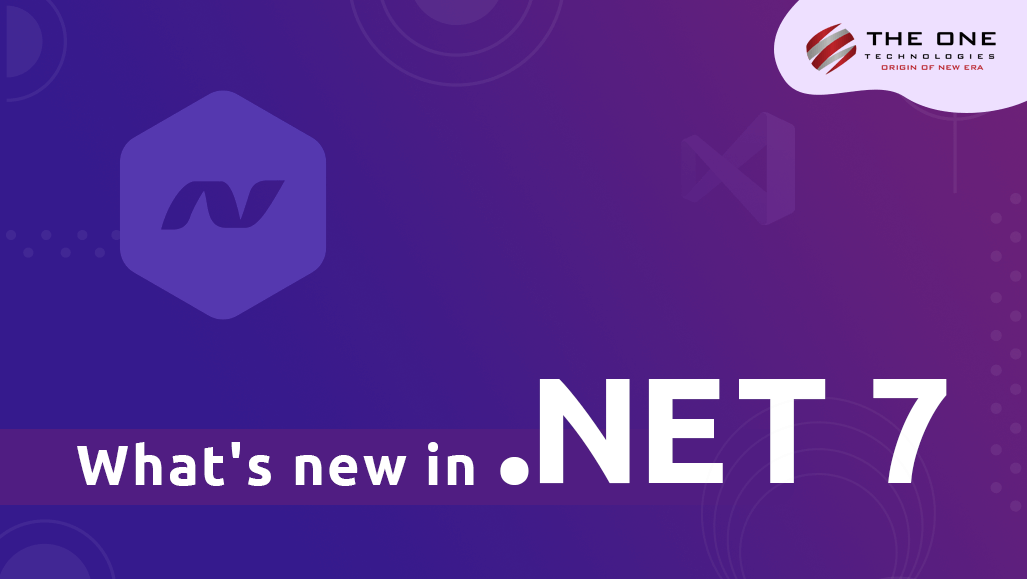
.NET 7 is the latest version of the .NET framework, released in November 2022. It brings several new features and improvements over previous versions, including:
Table of Contents
- Cross-platform support for Windows, Linux & macOS
- Improved performance and faster startup time
- Blazor WebAssembly, a new way to build web applications using .NET
- Support for building cloud-native apps with Azure Functions
- Improved support for C# 11 and .NET Native
- Enhanced support for .NET Core in Visual Studio
- Conclusion
Cross-platform support for Windows, Linux & macOS
Cross-platform support in .NET 7 means that developers can build applications using the .NET framework and run those applications on multiple operating systems, including Windows, Linux, and macOS. It is made possible through the use of .NET Core, the cross-platform implementation of the .NET framework.
Previously, developers had to write separate code for each operating system they wanted to support. With cross-platform support, they can write code a single time that can be run on any of the supported platforms. Developers can now quickly deploy their applications on different platforms without worrying about platform-specific differences.
In .NET 7, this cross-platform support has been improved further by introducing of a unified Base Class Library (BCL) and the development of .NET MAUI, a framework for building native applications for multiple platforms. These features make it even easier for developers to build applications that run seamlessly on various platforms.
BCL (Base Class Library) -
A .NET Framework library, BCL, is the standard for the C# runtime library and one of the Common Language Infrastructure (CLI) standard libraries. BCL provides types illustrating the built-in CLI data types, basic file access, collections, custom attributes, formatting, security attributes, I/O streams, string manipulation, and more.
.NET MAUI (Multi-platform App UI) -
.NET MAUI provides a unified development experience for creating modern, fast, and maintainable applications that run on Windows, macOS, iOS, and Android.
An example of a .NET MAUI application could be a cross-platform to-do list app that allows users to add, edit, and manage tasks. This app can be built using .NET MAUI and run natively on Windows, macOS, iOS, and Android devices. The user interface and app logic would be written in C# and shared across all platforms, while platform-specific code for things like UI styling or device capabilities could be added as needed. The result would be a single codebase that can run natively on multiple platforms, providing a seamless user experience to the end user.
Improved performance and faster startup time
Improved performance and faster startup time in .NET 7 can be demonstrated with the following examples:
(source: https://davecallan.com/net-6-v-net-7-reflection-performance-benchmarks/)
- Reduced memory usage: In .NET 7, the runtime has been optimized to reduce the memory footprint of applications. This results in faster and more efficient applications that use less memory, allowing them to run on systems with limited resources.
- Faster startup time: The startup time of applications built with .NET 7 has been improved by reducing the size of runtime and optimizing the initialization process. This means that applications will start up more quickly and be ready to use.
- Improved runtime performance: The runtime in .NET 7 has been optimized to improve the performance of applications. This includes improvements in the run time's ability to allocate and manage memory, as well as improvements to the garbage collector that reduce the time and frequency of pauses in application execution.
- Optimized for cloud and edge scenarios: .NET 7 has been designed with cloud and edge scenarios, as resource utilization is critical. The runtime and applications built with it are optimized for these environments, making them suitable for use in a wide range of deployment scenarios.
Cloud computing environments are defined by remote access to shared computing resources, while edge computing environments are defined by local processing at the edge of a network with limited resources. In both cases, resource utilization is critical, and applications must be efficient in their use of memory, processing power, and network bandwidth.
By being optimized for cloud and edge scenarios, .NET 7 makes it easier for developers to build and deploy applications in these environments, allowing them to take benefits of cloud and edge computing.
These improvements in .NET 7 result in applications that are faster, more responsive, and more efficient, leading to a better user experience.
Blazor WebAssembly, a new way to build web applications using .NET
Blazor WebAssembly is a new way of building web applications using .NET. It allows developers to write web applications using C# and .NET, rather than JavaScript. The applications run in the client's web browser and leverage WebAssembly, a low-level binary format for executing code in the browser.
Blazor WebAssembly provides a feature-rich platform for building web applications, including support for UI components, data binding, routing, and other common web development tasks. This makes it possible for .NET developers to build web applications with a familiar and well-documented programming language, tools, and libraries.
One of the key benefits of Blazor WebAssembly is that it enables full-stack .NET development, allowing developers to write both the client-side and server-side code for their web applications using .NET. This results in a more unstoppable development experience, as well as improved performance and security compared to traditional JavaScript-based web development.
Blazor WebAssembly is a new and exciting development in the world of web development and represents a significant opportunity for .NET developers to expand their skills and reach into new areas of application development.
-Example of Blazor WebAssembly would be a simple to-do list application. The application would have a user interface allowing the user to add and remove items from a list, which would be stored and displayed in the browser. Here's a some -outline of the code required to build the application:
- Add a UI component: This can be done by creating a Razor component, which is a combination of HTML, C#, and Razor syntax that defines the structure and behavior of the user interface. The component could be defined as follows -
<div>
<h3>To-Do List</h3>
<ul>
@foreach (var item in ToDo)
{
< li > @item </ li >
}
</ul>
<input type="text" @bind="newItemText" />
<button @onclick="AddItem"> Add </button>
</div>
Note - This is incomplete code, and may not run properly. Required further assistance from the experts.
- Write the code-behind: The code-behind is the C# code that provides the functionality of the component. It could be defined as follows-
private List<string> ToDo = new List<string>();
private string newItemText;
private void AddItem()
{
ToDo.Add(newItemText);
newItemText = string.Empty;
}
Note - This is incomplete code and may not run properly. Required further assistance from the experts.
- Implement data binding: Data binding is used to connect the user interface to the code behind it. In this example, the @bindattribute is used to bind the value of the input field to the newItemText property, and the@onclick attribute is used to bind the button click event to the AddItem method.
- Run the application: Once the code has been written, the application can be run in a browser, and the user interface will display the to-do list, allowing the user to add and remove items as they want.
This is just a simple example, but it demonstrates how Blazor WebAssembly can be used to build web applications using .NET.
Support for building cloud-native apps with Azure Functions
Support for building cloud-native apps with Azure Functions in .NET 7 refers to the capability to develop, deploy, and run Azure Functions using .NET 7, the latest version of the .NET platform. This support allows developers to leverage the features and capabilities of .NET 7 to build serverless, event-driven, and scalable applications in the cloud using Azure Functions. This includes features such as improved performance, enhanced security, and improved developer productivity, which makes it easier for developers to create cloud-native applications using Azure Functions and .NET 7.
Code Example 1 -
Function1.cs
using System;
using System.IO;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
namespace Function_1
{
public static class Function1
{
[FunctionName("Function1")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string name = req.Query["name"];
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
dynamic data = JsonConvert.DeserializeObject(requestBody);
name = name ?? data?.name;
string responseMessage = string.IsNullOrEmpty(name)
? "This HTTP triggered function executed successfully. Pass a name in the query string or in the request body for a personalized response."
: $"Hello, {name}. This HTTP triggered function executed successfully.";
return new OkObjectResult(responseMessage);
}
}
}
Output -
-With the support for building cloud-native apps with Azure Functions in .NET 7, developers can take advantage of the latest .NET platform features, such as improved performance, enhanced security, and productivity, to build scalable and efficient microservices. Additionally, Azure Functions provides a serverless computing environment, so developers don't need to worry about managing infrastructure and can instead focus on writing code.
Improved support for C# 11 and .NET Native
Improved support for C# 11 and .NET Native refers to the increased capabilities and features provided in the latest version of the C# programming language and .NET platform that allows developers to build more robust, efficient, and high-performance applications.
C# 11.0 is the latest version of the C# language and provides a number of new features and improvements, such as improved pattern matching, enhanced module support, and improved record types. With improved support for C# 9, developers can write more concise and readable code, making it easier to develop and maintain complex applications.
.NET Native is a technology that allows developers to compile .NET applications into native code for improved performance and efficiency. With improved support for .NET Native, developers can build faster and more responsive applications that take advantage of the full capabilities of the underlying hardware.
Together, improved support for C# 11 and .NET Native provides a more comprehensive development environment that enables developers to build high-performance and scalable applications more easily and efficiently.
Note - we will soon publish a blog on C#11.
Enhanced support for .NET Core in Visual Studio
Enhanced support for .NET Core in Visual Studio for using .NET 7 refers to the improved capabilities and features provided in Visual Studio, a popular integrated development environment (IDE), for developing .NET Core applications in the latest version of the .NET platform that is .NET 7.
With enhanced support for .NET Core in Visual Studio, developers can take advantage of a more comprehensive development environment that makes it easier to create code, debug code, and deploy code on a production or staging environment. .NET Core applications. This includes improved performance, enhanced debugging capabilities, and improved integration with other tools and services.
Additionally, the enhanced support for .NET Core in Visual Studio provides a more relatable development experience, allowing developers to focus on writing code and delivering business value rather than worrying about the underlying infrastructure and tooling. This results in increased productivity and a more efficient development process, making it easier for developers to create high-quality, scalable, and maintainable .NET Core applications.
Web application development for an eCommerce company is an example of enhanced support for .NET Core in Visual Studio through .NET 7. Using Visual Studio, the developers can create a .NET Core project, which allows them to take advantage of the enhanced support for .NET Core in the latest version of the .NET platform, .NET 7.
During development, the developers can use the improved debugging capabilities in Visual Studio to identify and fix bugs more easily. They can also take advantage of the improved performance to build a faster and more responsive web application.
Once the application is ready, the developers can use Visual Studio's improved integration with other tools and services to deploy the application to the cloud. They can also use Visual Studio's streamlined development experience to manage dependencies, build and test the application, and perform other common development tasks.
Conclusion
With the enhanced support for .NET Core in Visual Studio in .NET 7, the developers can focus on delivering business value and creating a high-quality web application without worrying about the underlying infrastructure and tooling. This results in increased productivity and a more efficient development process.
Overall, .NET 7 aims to provide a more cohesive and consistent experience for developers building modern applications across multiple platforms. It continues to evolve as a platform for building modern applications on Windows, macOS, and Linux. The features explained above are the new core features of .Net 7.
About Author
Anant Sirsath is a Jr. net developer. He started his career journey in January 2022 from The One Technologies - one of the best Net development company.
Till now, his journey has been challenging and good. He loves coding and traveling to explore new places. He learns new things during his leisure time