ASP.NET - login with facebook and graph API
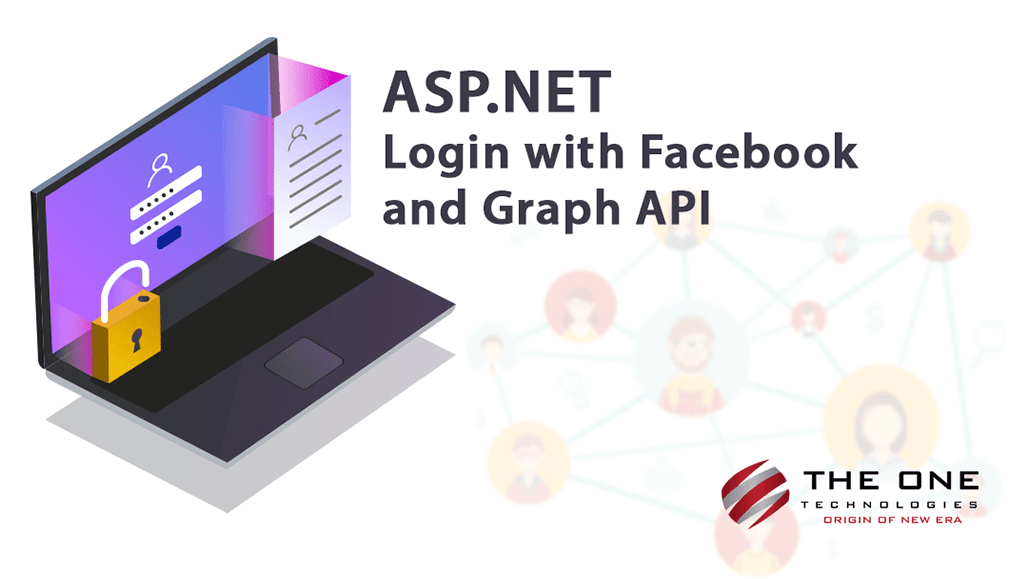
In this article, we will look at a simple web application, that can help you visualize the addition of Login By facebook functionality to your asp.net web application.
Facebook Application ID
It is mandatory to create a Facebook application on their developer portal and get the ApplicationId from there, which we can use in our code to authenticate user against facebook graph API.
We will be using Facebook Graph JSON responses in our code, so in order to deserialize the JSON string we need to create few class as follow:
public class FacebookUser
{
public long id{ get; set; }
public string email{ get; set; }
public string name
{ get; set; }
public string first_name
{ get; set; }
public string last_name
{ get; set; }
public string gender
{ get; set; }
public string link
{ get; set; }
public DateTime updated_time
{ get; set; }
public DateTime birthday
{ get; set; }
public string locale
{ get; set; }
public string picture
{ get; set; }
public FacebookLocation location
{ get; set; }
}
public class FacebookLocation
{
public string id
{ get; set; }
public string name
{ get; set; }
}
When user authenticate against Facebook, facebook will send Access Token, which we can use on behalf of user to make further requests to Facebook. We need to pages here:
- Front end page with Login link, which allows user to open Facebook Login dialog
- A callback page to which we redirect autheticated user to get additional information about user from Facebook.
Here is code for default.aspx page:
Code for callback.aspx
Here we are fetching user's additional details to display it (alternatively you can store it in your database). Please note the ApplicationID field here, which you need to replace with your own application Id.
public partial class callback : System.Web.UI.Page
{
//TODO: paste your facebook-app key here!!
public const string FaceBookAppKey = "XXXXXXXXXXXXX";
protected void Page_Load(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(Request.QueryString["access_token"])) return; //ERROR! No token returned from Facebook!!
//let's send an http-request to facebook using the token
string json = GetFacebookUserJSON(Request.QueryString["access_token"]);
//and Deserialize the JSON response
JavaScriptSerializer js = new JavaScriptSerializer();
FacebookUser oUser = js.Deserialize<FacebookUser>(json);
if (oUser != null)
{
Response.Write("Welcome, " + oUser.name);
Response.Write("<br />id, " + oUser.id);
Response.Write("<br />email, " + oUser.email);
Response.Write("<br />first_name, " + oUser.first_name);
Response.Write("<br />last_name, " + oUser.last_name);
Response.Write("<br />gender, " + oUser.gender);
Response.Write("<br />link, " + oUser.link);
Response.Write("<br />updated_time, " + oUser.updated_time);
Response.Write("<br />birthday, " + oUser.birthday);
Response.Write("<br />locale, " + oUser.locale);
Response.Write("<br />picture, " + oUser.picture);
if (oUser.location != null)
{
Response.Write("<br />locationid, " + oUser.location.id);
Response.Write("<br />location_name, " + oUser.location.name);
}
}
}
/// <summary>
/// sends http-request to Facebook and returns the response string
/// </summary>
private static string GetFacebookUserJSON(string access_token)
{
string url = string.Format("https://graph.facebook.com/me?access_token={0}&fields=email,name,first_name,last_name,link", access_token);
WebClient wc = new WebClient();
Stream data = wc.OpenRead(url);
StreamReader reader = new StreamReader(data);
string s = reader.ReadToEnd();
data.Close();
reader.Close();
return s;
}
}